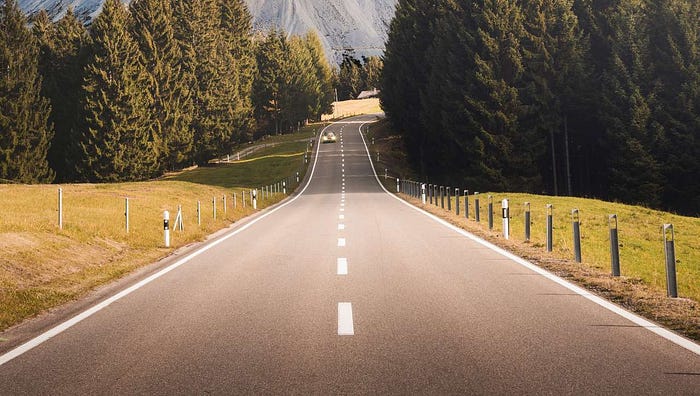
Keras Convolution layer — shapes of input, weights and output
Last Updated on April 7, 2024 by Editorial Team
Author(s): Sujeeth Kumaravel
Originally published on Towards AI.
Keras Convolution layer — shapes of input, weights and output
TensorFlow is one of the packages to develop solutions for machine learning problems. Keras is a module in TensorFlow that specifically offers tools to build neural network models.
Let us explore certain aspects of the convolution layer in Keras. In this tutorial let us focus on the 2D convolution layer.
Let us import Conv2D layer as follows.
from tensorflow.keras.layers import Conv2D
There are many arguments you can pass to create a Conv2D object. But let us give values for two arguments that are mandatory. They are
filters — int value specifying the number of 2D filters in the convolution
kernel_size — 2-tuple specifying the size of all the 2D filters. You can also pass an int value in which case, the filter is a square shaped one with kernel_size x kernel_size as dimensions
Let us create a Conv2D layer object as follows.
conv2d_1 = Conv2D(filters=32, kernel_size=(4, 4))
Here we have created a 2D convolution layer with 32 filters and 3 x 3 as the size of each of those 32 filters.
get_weights() method of a Keras layer object gives the weights of that layer. Let us see what the weights are for the Conv2D layer object created as follows.
conv2d_1.get_weights()
The output of this statement is as follows.
[]
You get an empty list. So, when you create a Keras layer object, initially it doesn’t have any weights even though you have specified the dimensions of the layer while creating it.
Now, let us load an image file named test_1.jpg which is the following:
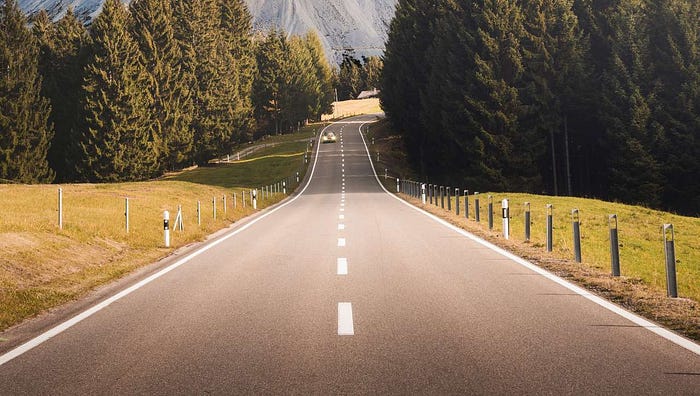
We upload this image as follows:
import cv2
img = cv2.imread('test_1.jpg')
The image gets loaded as a numpy array. Let us see the shape of this array as follows.
img.shape
We get the shape as
(725, 1280, 3)
It is an RGB image and so we have 3 channels. Now let us process this images using the 2D convolution layer we just created. Before that, let us take this single image into a batch of size 1 as follows. This is because, Keras layers take batches of data samples (images in this case) as input.
import numpy as np
img_batch = np.array([img])
Let us see what the shape of img_batch is
img_batch.shape
The shape we get is
(1, 725, 1280, 3)
We see that a batch of size 1 is created (the image we uploaded is the single data sample in the batch).
The specific image test_1.jpg we uploaded has its pixel values as uint8 data type.
img_batch.dtype
Output of this statement is
dtype('uint8')
But, Conv2D layer in Keras doesn’t process uint8 data. So, let us convert the image numpy array into float data type as follows.
img_batch = img_batch.astype('float')
Let us see what the data type now is
img_batch.dtype
Output of this statement is
dtype('float64')
Now we have typecast our batch of image data into float datatype. Let us process our image batch using the 2D convolution layer we have created as follows.
y = conv2d_1(img_batch)
The 2D convolution layer object should be called by passing the image batch as argument. Earlier we saw that a Keras layer objectdoesn’t have weights after getting created. But, when you call it by passing in a data argument, the weights get initialized. We can get these initialized weights using the get_weights() method of the layer object as follows.
w = conv2d_1.get_weights()
Let’s see what is in the weights variable w. First let us see what the type of w is.
type(w)
We get the following output.
list
So, the weights variable is a list. Let us see how many elements are in this list as follows.
len(w)
We get
2
There are two elements in the weights list. Let us see what the type of data is in each of these two elements.
type(w[0])
We get
numpy.ndarray
The type of the second element in the weights list is
type(w[1])
We get
numpy.ndarray
The two elements in the weights list are numpy arrays. Let us see what the shape of each of these two numpy arrays in the weights list is.
w[0].shape
We get
(4, 4, 3, 32)
How to interpret this shape? The input image has 3 channels. We have created the convolution layer to have 32 filters, each of dimension 4 x 4. So, the first 2 elements denote the filter size, the third element denotes the number of channels in the input data and the final element denotes the number of filters in the layer.
Let us see the shape of the second element in the weights list.
w[1].shape
We get:
(32,)
We get a one dimensional numpy array with the length equal to the number of filters. The first element in the weights list contains the weights of each of the 32 filters of shape 4 x 4 x 3. The second element in the weights list correspond to the bias of the filters. There is one bias for each of the 32 filters in the layer.
Let us take a sneak into the values in the two elements in the weights list.
Values in w[0]:
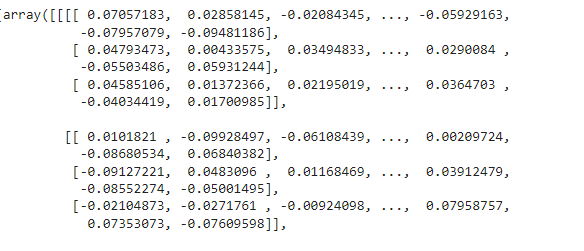
The dtype of this array is float32.
Values in w[1]:
array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.,
0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.],
dtype=float32)
Let us see the shape of the output of the Conv2D layer as follows.
y.shape
We get:
TensorShape([1, 722, 1277, 32])
So, the output is a tensor. In convolution operation between two arrays — one of size N and another of size M — the output’s size will be N — M + 1. The height dimension of the image was 725 and that of the filter was 4. So, the height of the output = 725–4 + 1 = 722. Similar reason applies to the width dimension. The number of channels in the output is 32 which is the number of filters.
With this I conclude a basic insight into how a convolution layer in Keras processes data.
Signing off now!
References:
Keras documentation: Conv2D layer
Keras documentation
: Conv2D layer
Keras documentationkeras.io
Join thousands of data leaders on the AI newsletter. Join over 80,000 subscribers and keep up to date with the latest developments in AI. From research to projects and ideas. If you are building an AI startup, an AI-related product, or a service, we invite you to consider becoming a sponsor.
Published via Towards AI