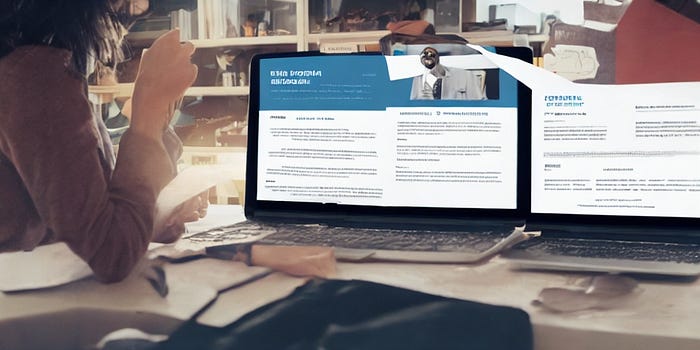
The Future of Resumes: Craft Your Interactive Resume Web App
Last Updated on December 30, 2023 by Editorial Team
Author(s): Muhammad Saad Uddin
Originally published on Towards AI.
Welcome to the digital age, where paper resumes are as outdated as dial-up internet! If you’re still sending out PDF resumes, I hate to break it to you, but that’s about as cutting edge as a butter knife. Today’s recruiters are on the hunt for candidates who can make a memorable first impression. Sure, you could do that with a scented resume, but we’re not in a rom-com movie. So, let’s dive into the AI-accelerated century and create something unique and interactive— a web app resume!
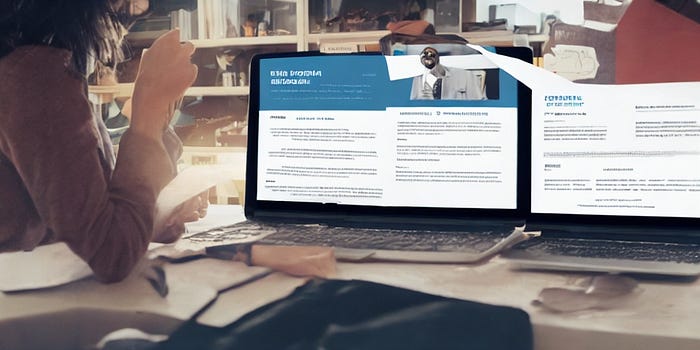
In this riveting guide, I’ll show you how to transform your drab resume into an interactive web app, using Python and Streamlit. This will not only make your profile stand out, but it also allows potential employers to see your tech skills in action. It’s like killing two birds with one stone, except no birds were harmed in the making of this tutorial. By the end of this journey, you’ll have a resume that’s not just a piece of paper (or rather a PDF file) but an immersive experience. So, get ready to code your way to your dream job!
Getting Started: Required Tools and Libraries
Let’s not beat around the bush, you’re itching to get started. But before we start weaving code into a tapestry of interactive resume brilliance, we need the right tools. It’s like wanting to paint a masterpiece but not having a brush. Or wanting to bake a cake without an oven. Or wanting to…you get the idea.
Our magic wand in this journey is Python — the world’s favorite snake after Kaa from The Jungle Book. Python is loved for its simplicity and power, making it the perfect choice for our task. Next up, we need Streamlit. Streamlit is a library that lets us create web applications with Python. Yes, you heard it right! No need to juggle between HTML, CSS, and JavaScript. Streamlit will take care of all the heavy lifting, so you can focus on what truly matters — creating an impressive resume. Then there’s Plotly, our handy tool for creating beautiful visualizations. We’ll use it to plot bar charts and pie charts to represent your skills and competencies. It’s like the cherry on top of our interactive resume cake.
pip install streamlit plotly
import streamlit as st
import streamlit.components.v1 as components
import plotly.graph_objs as go
import plotly.express as px
Once you’ve got Python, Streamlit, and Plotly installed and ready, we can dive right into creating our interactive resume. Strap in, it’s going to be quite a ride!
Setting Up the Web App Interface
Alright, we’re all set with our tools, so it’s time to roll up our sleeves and get our hands dirty (virtually speaking). Now, if you’ve ever tried to build a sandcastle with no plan in mind, you’ll know that the first step to creating something impressive is to start with a blueprint. So, let’s outline our web app interface.
Imagine walking into a bookstore. You don’t just find books strewn around haphazardly. There’s an order, a method to the madness. Similarly, our web app needs a structure. Streamlit provides two main areas where we can display our content — the sidebar and the main page. Think of the sidebar as the table of contents of a book. It’s where we can place navigation controls, filters or a delightful little chatbot to answer queries about your professional life. It’s like your personal assistant, guiding visitors through your digital resume.
The main page, on the other hand, is the heart of our web app. It’s where the meat of the content goes. This is where we’ll showcase your about me section, work experience, education, projects, skills, and certifications. It’s like the chapters of a book, each unfolding a new aspect of your professional journey.
To set up the interface, we’ll use Streamlit’s functions, mainly st.sidebar
for the sidebar and st
for the main page. Streamlit also provides a st.set_page_config
function to configure the page title and layout.
def main():
st.set_page_config(page_title="Saad's Resume", page_icon=":memo:", \
layout="wide")
st.sidebar.write("Toggle Checkbox for a Chat")
if st.sidebar.checkbox("Ask Me! via Chat"):
st.sidebar.write("Chatbot [Not a LLM yet]")
user_name = st.sidebar.text_input("What should I call you?")
user_company = st.sidebar.text_input("Your Company")
if user_name and user_company:
st.sidebar.write(f"Hello, {user_name}! Glad to know you work for \
{user_company}.")
questions = [ "Add your questions/ multiple questions " ]
selected_question = st.sidebar.selectbox("Choose a question", questions)
if st.sidebar.button("Ask"):
if selected_question == questions[0]:
st.sidebar.write(" your answer ")
elif selected_question == questions[1]:
st.sidebar.write(" your second answer ")
#add more based on your style and ideas
As shown in the code, I have added a chatbot in the sidebar just for basic interaction. Now, hold on to your hats because we’re about to dive into the detail code and bring our web app to life. Remember, every great journey starts with a single step, or in our case, a single line of code.
Crafting Your Professional Story: About Me, Experience, and Education
Now that we’ve set up our digital canvas, it’s time to paint our masterpiece. Our colors? Lines of code. Our brush strokes? Functions and methods. Our subject? You.
Let’s start by introducing the star of our show — you. The ‘About Me’ section is where you get to share your unique story. It’s where you get to show your personality, your passion, and your aspirations. This is not just about stating facts, it’s about telling a story. We’ll use a combination of Markdown and HTML to add a profile picture and a captivating introduction about yourself. Remember, first impressions matter, so let’s make this count!
# Profile picture and About Me section
profile_picture = "xyz.png"
about_me_bg_color = "#f0f0f0"
about_me_text_color = "#333333"
about_me_styles = f"""
<style>
.about_me {{
background-color: {about_me_bg_color};
color: {about_me_text_color};
padding: 1rem;
margin-bottom: 1rem;
border-radius: 10px;
}}
</style>
"""
st.markdown(about_me_styles, unsafe_allow_html=True)
col1, col2 = st.columns([1, 3])
with col1:
st.image(profile_picture) #, width=250
with col2:
st.markdown('<div class="about_me">', unsafe_allow_html=True)
st.header("My Name")
st.subheader("My Headline")
st.write("A description about me")
st.markdown('</div>', unsafe_allow_html=True)
#st.markdown("---")
st.markdown("# Personal Information")
#st.markdown("---")
col1a, col2a = st.columns([1, 1])
with col1a:
# Phone
st.markdown(" Phone is not available as this is a public site")
# Email
st.markdown(" Email is not available for the same reason")
# Website
st.markdown("[](my webpage) But you can visit my webpage by clicking on icon")
#coursera link
st.markdown("[](profile) My Instructor Profile @ Coursera, click on icon")
with col2a:
# LinkedIn
st.markdown("[](linkedIn) Click on icon to see my LinkedIn")
# GitHub
st.markdown("[](github link) Explore my GitHub by clicking on icon")
# Medium
st.markdown("[](medium link) Follow me on Medium for latest Development in AI by clicking on icon")
#Credily link
st.markdown("[](Verifications) Verify my achievements at Credly by clicking on icon")
In this code block, We first set variables for the profile picture and colors for the ‘About Me’ section. The profile_picture
variable holds the file name of the picture, while about_me_bg_color
and about_me_text_color
hold the background color and text color for the 'About Me' section. We then define the CSS styles for the ‘About Me’ section using the colors set in the previous step. The about_me_styles
string holds the CSS code for this.
Next, we use the st.columns
function to divide the page into two columns. The first column will hold the profile picture, and the second will hold the 'About Me' section.
In the first column, we use the st.image
function to display the profile picture. In the second column, we create the ‘About Me’ section. We first open a div with the class ‘about_me’, which applies the styles we defined earlier. We then add the name, headline, and description. After the ‘About Me’ section, we create a ‘Personal Information’ section. We again divide the page into two columns. In the first column of the ‘Personal Information’ section, we add the phone, email, and website information. We use the st.markdown
function to add these details, and use markdown to add images and links. In the second column of the ‘Personal Information’ section, we add links to LinkedIn, GitHub, Medium, and Credly. We use the st.markdown
function again to add these links, and use markdown to add images and links.
#below is the code within main()
st.write(" ")
st.write(" ")
# Work experience with interactive timeline
st.markdown("---")
st.header("Work Experience")
#st.markdown("---")
year = st.slider("Select a year:", min_value=2017, max_value=2023, value=2023, step=1)
display_work_experience(year)
st.write(" ") #wrk should be before education
st.write(" ")
st.write("---")
st.header("Education")
#st.markdown("---")
st.subheader("My education 1")
st.write("detail")
st.write("Specialization:")
st.write(" ")
st.subheader("My education 2")
st.write("")
st.write("")
# we define functions outside of main() organization, reusability and modularity
def display_work_experience(year):
if year <= 2019:
st.subheader("Position 1")
st.write("- point 1")
st.write("- point 2")
elif year > 2019 and year < 2021:
st.subheader("Position 2")
st.write("- point 1")
st.write("- point 2")
## add more based on your experience
We do the same for the ‘Work Experience’ & ‘Education’ sections. To make the experience section interactive, we’ll use a slider to display different work experiences based on the year selected. As we build these sections, remember, our aim is not just to share information but to engage, to captivate, and to impress. So let’s get coding and craft a professional story that’s as unique as you are!”
Showcasing Your Expertise: Projects, Skills, and Certifications
Having introduced the star of our show and taken our audience on a journey through your professional and academic life, it’s time to pull out the big guns — your projects, skills, and certifications. This is where we prove that you’re not just all talk, but you’ve got the chops to back it up.
First up, we have the ‘Projects’ section, where we highlight your hands-on experience. This isn’t just a list of project titles. Oh no, we’re going to make it interactive. Each project will be presented in an expandable format, containing a brief description of the technologies used and your role in it. But we won’t stop there. We’ll also create bar charts to visualize the skills used in each project. It’s like opening a box to reveal the treasure inside
#outside of main()
def create_horizontal_bar_chart(data, title):
fig = px.bar(data, x="Percentage", y="Skill", orientation="h")
fig.update_layout(title_text=title, showlegend=False)
return fig
def display_project_details(project): #use a dict to hold all projects
data_dict = {
'Project 1: Name':['Description']
'Project 2: Name':['Description']
}
#add more
chart_dict = {
'Project 1: Name':[['Skills'], ['Score']]
}
details_list = data_dict[project]
plot_list = chart_dict[project]
datap = {"Skill": plot_list[0], "Percentage": plot_list[1]}
if len(details_list) <= 1:
cold,cole = st.columns(2)
with cold:
st.subheader("Description:")
st.write(details_list[0])
with cole:
chart1 = create_horizontal_bar_chart(datap, "Skills Used")
st.plotly_chart(chart1, config={"displayModeBar": False}, use_container_width=True)
if len(details_list) > 1:
cold,cole = st.columns(2)
with cold:
st.subheader("Description:")
st.write(details_list[0])
chart1 = create_horizontal_bar_chart(datap, "Skills Used")
st.plotly_chart(chart1, config={"displayModeBar": False}, use_container_width=True)
with cole:
st.image(details_list[1])
st.image(details_list[2])
st.image(details_list[3])
#part of main() funciton
st.markdown("---")
st.header("Projects")
#st.markdown("---")
projects = [
"Select a project",
"Project 1: Name",
"Project 2: Name",
#add more
]
selected_project = st.selectbox("Click on a project to see more details:", projects)
if selected_project != "Select a project":
with st.expander("Click here to expand and learn more about this project roject"):
display_project_details(selected_project)
create_horizontal_bar_chart(data, title)
: This function creates a horizontal bar chart using Plotly Express. It takes in a data dictionary and a title for the chart, and returns a Plotly figure.display_project_details(project)
: This function displays the details of a selected project. It uses two dictionaries:data_dict
holds the descriptions of the projects andchart_dict
holds the skills used and their scores. The function creates a bar chart of the skills used in the project and displays the project description. If there are more than one detail elements, it also displays additional images.- It first adds a header “Projects” to the webpage. It then creates a dropdown list of projects using
st.selectbox
. Users can select a project from this list to view its details. If a project is selected (i.e., the selected project is not “Select a project”), it usesst.expander
to create a section that can be expanded to show the details of the project. It calls thedisplay_project_details
function to display the details of the selected project.
Next, we move on to ‘Skills’. Now, this isn’t just a laundry list of skills you possess. That’s too mundane for our interactive resume. Instead, we’ll represent your skill proficiency using pie charts. It’s a visual and interactive way to show not just what skills you have, but how well you’ve mastered them.
#part of main() funciton
st.markdown("---")
st.markdown("# Skills")
st.write("Click on a skill to expand details")
st.markdown("---")
skills = [
(" Python", Score, "Description", "Icon"),
(" Data Science", Score, "Description", "Icon"),
#add more skills
]
# Number of buttons per row
num_buttons_per_row = 8
# Calculate the number of rows required
num_rows = -(-len(skills) // num_buttons_per_row)
# Create buttons and display charts upon clicking
selected_skill = None
for i in range(num_rows):
cols = st.columns(num_buttons_per_row)
for j in range(num_buttons_per_row):
idx = i * num_buttons_per_row + j
if idx < len(skills):
skill, proficiency, description, icon = skills[idx]
if cols[j].button(skill):
selected_skill = skill, proficiency, description, icon
if selected_skill:
skill, proficiency, description, icon = selected_skill
fig, desc = create_skill_chart(skill, proficiency, description, icon)
cola1, cola2 = st.columns(2)
with cola1:
st.plotly_chart(fig)
with cola2:
st.write(" ")
st.subheader(desc)
#outside of main()
def create_skill_chart(skill, proficiency, description, icon):
fig = go.Figure(go.Pie(
values=[proficiency, 100 - proficiency],
labels=[f"({proficiency}%)", ""],
hole=0.6,
textinfo='label',
hoverinfo='none'
))
fig.update_traces(marker=dict(colors=['blue', 'white']))
fig.add_annotation(
go.layout.Annotation(
text=f"<b>{skill}</b>",
x=0.5,
y=0.5,
showarrow=False,
font=dict(size=24),
align='center',
valign='middle'
)
)
fig.update_layout(showlegend=False)
return fig, description
create_skill_chart(skill, proficiency, description, icon)
: This function creates a pie chart that shows the proficiency of a skill using Plotly's graph objects. The chart shows proficiency as a percentage of a whole (100). The function also adds an annotation in the middle of the pie chart, which is the name of the skill. The function returns the figure and the skill's description.- This code creates a grid of buttons for different skills. When a button is clicked, it displays a pie chart that shows the proficiency level of the corresponding skill and a description of the skill. It uses Streamlit’s interactive features and Plotly’s charting capabilities to create an interactive skills section in a web app.
Finally, we have the ‘Certifications’ section. This isn’t just a space to list your certifications. It’s a place to showcase your dedication to continuous learning and professional growth. Here, we’ll display linked images of your certifications. One click, and your potential employer can see your certification proof. It’s like giving them a VIP tour of your accomplishments.
st.markdown("---")
st.write("## Certifications")
st.write("Click on a certification to expand details")
Certifications = [
("Name", "Img link", ["Skills"], [Score]),
("Name", "Img link", ["Skills"], [Score]),
#add more
]
# Number of buttons per row
num_buttons_per_row1 = 4
# Calculate the number of rows required
num_rows1 = -(-len(Certifications) // num_buttons_per_row1)
# Create buttons and display charts upon clicking
selected_skill1 = None
for i in range(num_rows1):
colss = st.columns(num_buttons_per_row1)
for j in range(num_buttons_per_row1):
idx1 = i * num_buttons_per_row1 + j
if idx1 < len(Certifications):
certi, link, skil, perc = Certifications[idx1]
if colss[j].button(certi):
selected_skill1 = certi, link, skil, perc
if selected_skill1:
certi, link, skil, perc = selected_skill1
data = {"Skill": skil, "Percentage": perc}
chart = create_horizontal_bar_chart(data, "Skills Learned")
cold1, cold2 = st.columns(2)
with cold1:
st.image(link)
with cold2:
st.plotly_chart(chart, config={"displayModeBar": False})
if __name__ == "__main__":
main()
- This code creates a grid of buttons for different certifications. When a button is clicked, it calls the
create_horizontal_bar_chart
function to create the bar chart for the skills learned in the selected certification. It then displays the certification image and the bar chart in two columns.
Remember, our goal is to make your resume not just informative, but interactive and engaging. So, let’s dive in and showcase your expertise in a way that leaves a lasting impression.
Launching and Sharing Your Interactive Resume Web App
Alright, the moment of truth has arrived. It’s time to take our interactive resume web app for a spin. But we won’t stop at just running it locally. Oh no, we’re going to share it with the world. It’s like hosting your own digital exhibition, and your resume is the star attraction.
Running the web app locally is a breeze. All you need is to navigate to your project directory in your terminal and execute the following command:
streamlit run your_app.py
Voila! Your web app is up and running on your local machine. It’s like having a private viewing of your masterpiece. But why stop there?
The true power of a web app lies in its accessibility. So, let’s deploy it on a server for public access. Streamlit offers its own hosting platform, the Streamlit Community Cloud, which allows you to deploy your app with just one click. Before we begin, ensure your app and all its dependencies are available on a GitHub repository dedicated to this project. Your directory structure should look like this:
your-repository/
├── your_app.py
└── requirements.txt
If you have any custom configuration or theming, your config file should be named .streamlit/config.toml
and saved relative to the root of your repo.
Here’s how to deploy your app:
- Navigate to your workspace at share.streamlit.io.
- Click “New app” in the upper-right corner of your workspace.
- Fill in your repo, branch, and file path. You can also click “Paste GitHub URL” to paste a link directly to
your_app.py
on GitHub. - An app URL with a random hash is prefilled, but you can change this to a custom subdomain instead. Your app would then be deployed to
https://<your-custom-subdomain>.streamlit.app/
.
If you are still confused, this Streamlit document would be quite helpful: Steamlit: Deploy your App
Now that you’re familiar with the deployment process, let’s take a look at the final product. Here is a GIF showing how the web app looks and functions:
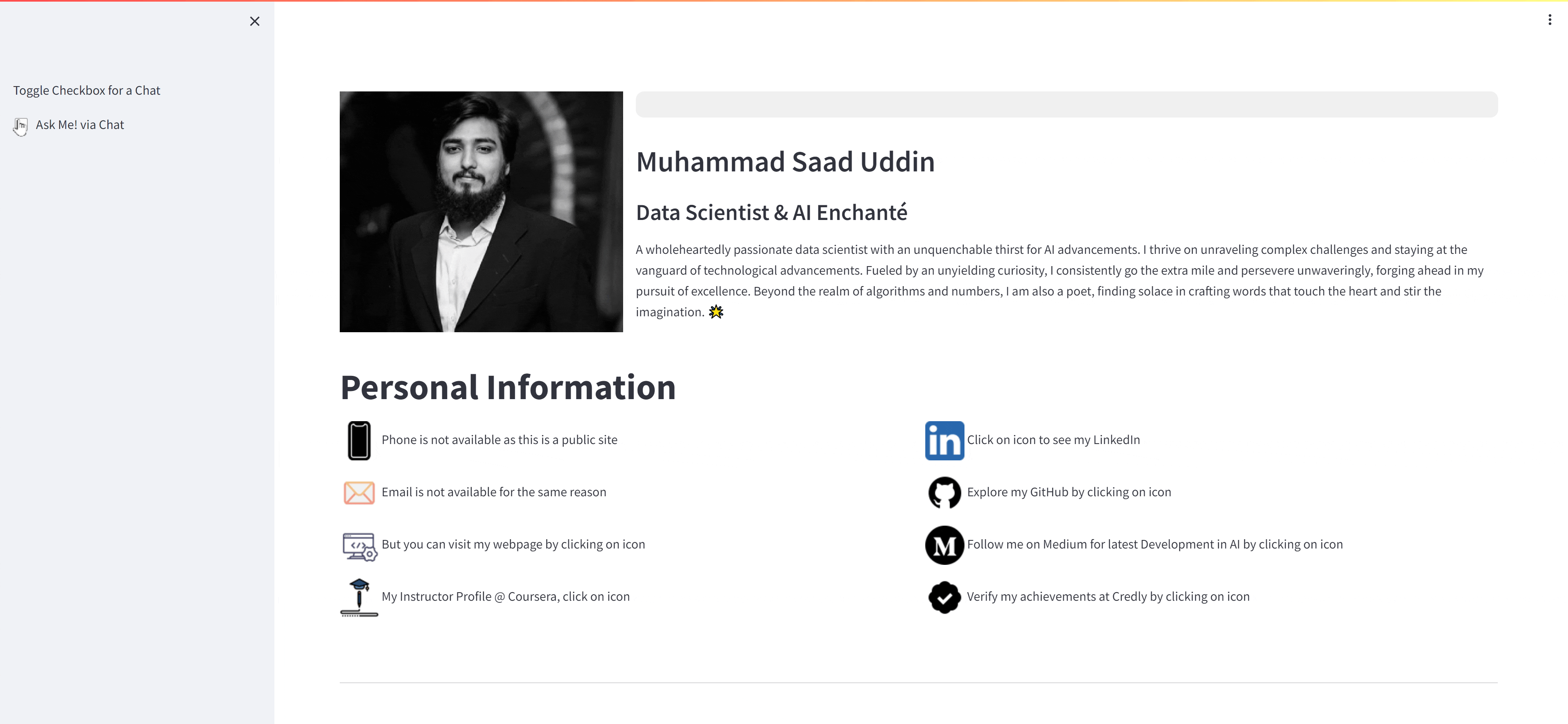
and you can visit my webpage here: https://saadresume.streamlit.app/
And for those interested in examining the complete code, you can find it in this GitHub repository:
So go ahead, launch your interactive resume web app. Let your unique professional story be heard, or rather, experienced. After all, your journey deserves to be shared.
Wrapping Up, Overcoming Hurdles, and Sharing Your Masterpiece
As we pull the curtain down on our coding saga, it’s time to sit back, take a deep breath, and admire the interactive web app resume we’ve created. From setting up our Python environment, outlining the web app interface, crafting your professional story, to showcasing your certifications; every step was a significant stride towards creating a resume that’s truly you.
We’ve turned a typically static document into a dynamic, interactive experience. You now have a resume that doesn’t just state facts, but tells your story, displays your journey, and highlights your achievements in a way that’s engaging, compelling, and downright impressive. And remember, this is your web app — tailor it, tweak it, make it truly yours. You’ve got the reins, so take charge and make your resume a reflection of who you are as a professional. But of course, every journey has its bumps. Coding is no different. As you develop and customize your resume, you might encounter some unexpected issues. But fear not, for every problem, there’s a solution. In this final section, we’ll discuss common issues that might crop up and how to overcome them. Consider it your troubleshooting map, guiding you through the sometimes confusing terrain of code.
And now, the most exciting part U+1F38A — sharing your masterpiece with the world. I’d love to see the fantastic resumes you’ve created. So, don’t forget to share them in the comments section below. Your journey could be the inspiration for many others. So, here’s to you, the creator of your interactive resume, the storyteller of your professional journey. Take this knowledge, create, innovate, and, most importantly, make your mark. After all, the world is but a canvas to our imagination.
If you found this guide helpful and wish to explore more exciting projects together, make sure to follow me. With each new project, I promise a journey filled with learning, creativity, and fun. Furthermore:
- U+1F44F Clap for the story (50 claps) to help this article be featured
- U+1F514 Follow Me: LinkedIn U+007CMedium U+007C GitHub
Join thousands of data leaders on the AI newsletter. Join over 80,000 subscribers and keep up to date with the latest developments in AI. From research to projects and ideas. If you are building an AI startup, an AI-related product, or a service, we invite you to consider becoming a sponsor.
Published via Towards AI