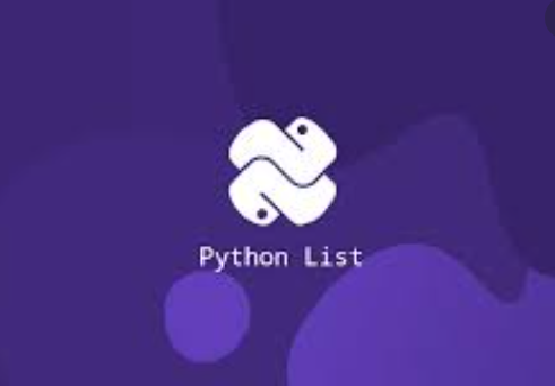
Python’s List Comprehensions
Last Updated on July 20, 2023 by Editorial Team
Author(s): Vivek Chaudhary
Originally published on Towards AI.
Programming
Lists are Python Data structures that are used to store multiple elements in a single variable. List comprehension is a more simple way to define and create a list in python, lists can be created in one line.
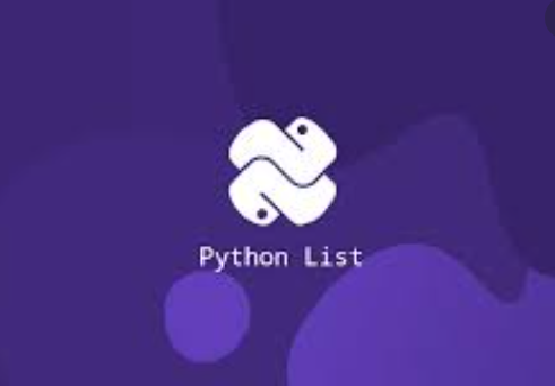
The syntax of list comprehension is easier to grasp as well.
The structure of a List comprehension program is below:
- An expression, which will be the output list
- An Input sequence
- A variable representing a member of the input sequence and
- An optional predicate part, where we can define our conditional statements
There can be multiple ways of performing a task in any programming language, similarly with python list comprehensions as well.
In my article we will straightaway not use list comprehension but will study the concept in a relational way, comparing it with loops, and then conclude the study, as I personally found this way a lot easier to understand.
Syntax of List Comprehension:
[expression for <item> in <list>]
Understanding syntax in details:
e.g [i**2 for i in range(100) if i%2==0 and i%5==0]
i**2 → is expression
for i in range(100) → input sequence and i is the variable
if i%2==0 and i%5==0 → literals and its an optional part
In the below examples, we will develop a better understanding of the concept.
1. Generate a list of sequential numeric items, starting from 1 to 10.
#generate sequence of numbers
my_list=[i for i in range(10)]
print(my_list)Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]

#Note: Just a single line code using comprehensions
2. Square of List Items.
#squaring list items
my_list=[5,4,8,7,2,9]
new_list=[i*i for i in my_list]
print(new_list)Output: [25, 16, 64, 49, 4, 81]

3. List comprehension with conditionals, create a new list of even numbers:
#if-else in list comprehension
my_list=[5,4,8,7,2,9]
new_list=[i for i in my_list if i%2==0]
print(new_list)Output: [4, 8, 2]

4. List Comprehension with Nested IF-ELSE
#nested if-else with comprehensions
new_list=[i for i in range(100) if i%2==0 and i%5==0]
print(new_list)Output: [0, 10, 20, 30, 40, 50, 60, 70, 80, 90]

5. Create a List of Square of Odd numbers.
odd_sqr=[i**2 for i in range(10) if i%2!=0]
print(odd_sqr)Output: [1, 9, 25, 49, 81]

6. Nested List comprehension.
create a list of lists using nested loops and list comprehensions:
#nested comprehension
l=[[j for j in range(5)] for i in range(5)]
print('comprehension output ',l)#looping over list items for understanding
for i in range(0,len(l)):
print('list items @ position '+' '+str(i)+' '+str(l[i]))
In nested list comprehensions, inner loop passes value to the outer loop, in above example value of i is passed to j in every iteration.

Summary
· List Comprehension Syntax with basic example
· List Comprehension with IF-ELSE
· List Comprehension with Nested If-Else
· List Comprehension to create squares of odd-even numbers
Thanks for reading my story. If you like my work do appreciate the efforts it encourages to create more quality stuff. Do connect with me on medium, share the content to spread the knowledge.
Join thousands of data leaders on the AI newsletter. Join over 80,000 subscribers and keep up to date with the latest developments in AI. From research to projects and ideas. If you are building an AI startup, an AI-related product, or a service, we invite you to consider becoming a sponsor.
Published via Towards AI