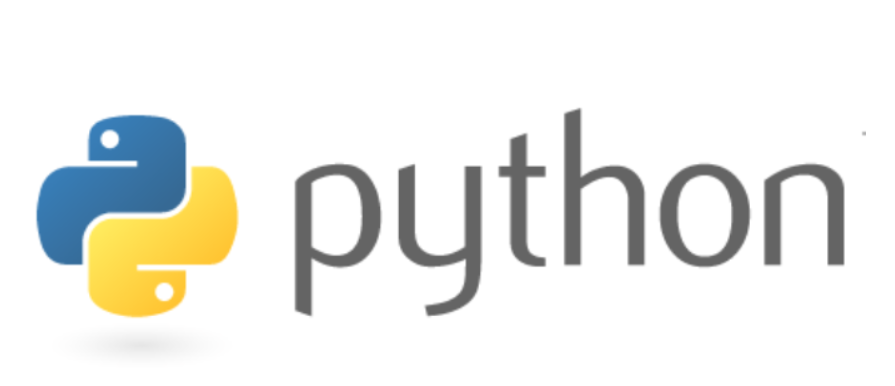
Count python list item occurrences
Last Updated on July 17, 2021 by Editorial Team
Author(s): Vivek Chaudhary
Programming
From a list of integers, count the occurrence of each element and add items and count as a sub-list.
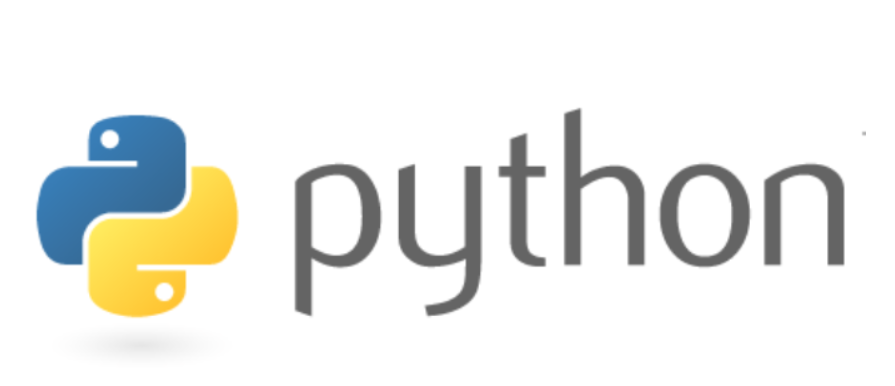
Input list: inp = [7, 9, 7, 4, 3, 5, 3, 6, 9, 3]
Output list: out= [[7, 2], [9, 2], [4, 1], [3, 3], [5, 1], [6, 1]]
#Note: where 7 is list item and 2 is the count of occurrence
Using nested loops
def count_items(inp):
for i in range(0, len(inp)):
a = 0
row =[]
if i not in l:
for j in range(0, len(inp)):
if inp[i]== inp[j]:
a = a + 1
row.append(inp[i])
row.append(a)
l.append(row)
for j in l:
if j not in out:
out.append(j)
return out
Call the function and check the output:
#call func()
inp = [7, 9, 7, 4, 3, 5, 3, 6, 9, 3]
l = []
out = []
print(count_items(inp))
Output: [[7, 2], [9, 2], [4, 1], [3, 3], [5, 1], [6, 1]]
Using count() method
def count_items(inp):
for i in inp:
row =[]
ct = 0
ct = inp.count(i)
row.append(i)
row.append(ct)
l.append(row)
for j in l:
if j not in out:
out.append(j)
return out
Call the function and check the output:
#call func()
inp = [7, 9, 7, 4, 3, 5, 3, 6, 9, 3]
l = []
out = []
print(count_items(inp))
Output: [[7, 2], [9, 2], [4, 1], [3, 3], [5, 1], [6, 1]]
Using counter() method
from collections import Counter
def count_items(inp):
coll = Counter(inp)
out = []
for key,val in coll.items():
out.append([key,val])
return out
Call the function and check the output:
#call func()
inp = [7, 9, 7, 4, 3, 5, 3, 6, 9, 3]
print(count_items(inp))
Output: [[7, 2], [9, 2], [4, 1], [3, 3], [5, 1], [6, 1]]
To summarize, we covered several ways to count occurrences of list items as below:
- count() method
- nested for loops
- collections library counter() method
That’s all with count occurrences of list items.
Thanks for reading my blog and supporting the content. Appreciation always helps to keep up the spirit. Will try my best to keep coming up with high-quality content. Connect with me to get updates about upcoming new content.
Count python list item occurrences was originally published in Towards AI on Medium, where people are continuing the conversation by highlighting and responding to this story.
Published via Towards AI